Position dependent rotation
Nebula 14 Agenda: “It encrypts input and writes it to standard output. An encrypted token file is also in that home directory, decrypt it :)”
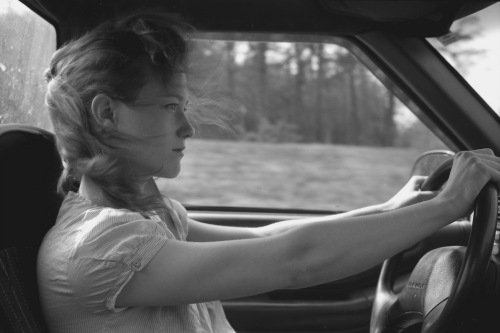
During the investigation under the hood, it’s was easy to recognize position dependent ROT (means, not 13 =). In current case, each char’s shift is directly proportional to its position in the sequence):
1 level14@nebula:/home/flag14$ ./flag14 -e
2 111111111
3 123456789
4 level14@nebula:/home/flag14$ ./flag14 -e
5 aaaaaaaaa
6 abcdefghi
Thus it was easy to write appropriate decoder:
1 #!/usr/bin/python
2 import sys
3
4 def decode(string):
5 if string is not None:
6 result = ""
7 for i in range(0, len(string)):
8 result += chr(ord(string[i]) - i)
9 return result
10
11
12 def readContentOf(filename):
13 try:
14 with open (filename, "r") as tokenFile:
15 return tokenFile.read().replace('\n', '')
16 except IOError:
17 sys.stderr.write('Problem reading:' + filename)
18
19
20 def main():
21 if (len(sys.argv) >= 2) :
22 text = readContentOf(sys.argv[1])
23 print "Your token is: ", decode(text)
24 else :
25 print "Please specify the toke_file"
26
27
28 if __name__ == "__main__":
29 main()
And obtain the token:
1 level14@nebula:~$ ./decoder.py /home/flag14/token
2 Your token is: 8457c118-887c-4e40-a5a6-33a25353165
Resources: